Get started with Vert.x
In this guide, you’ll learn how to get started with a new Vert.x Web project.
Before starting, you need:
- JDK 1.8 or higher
- A text editor or IDE
- Maven 3 or higher
- curl or HTTPie or a browser to perform HTTP requests
1Bootstrap
To create a new project, go to start.vertx.io.
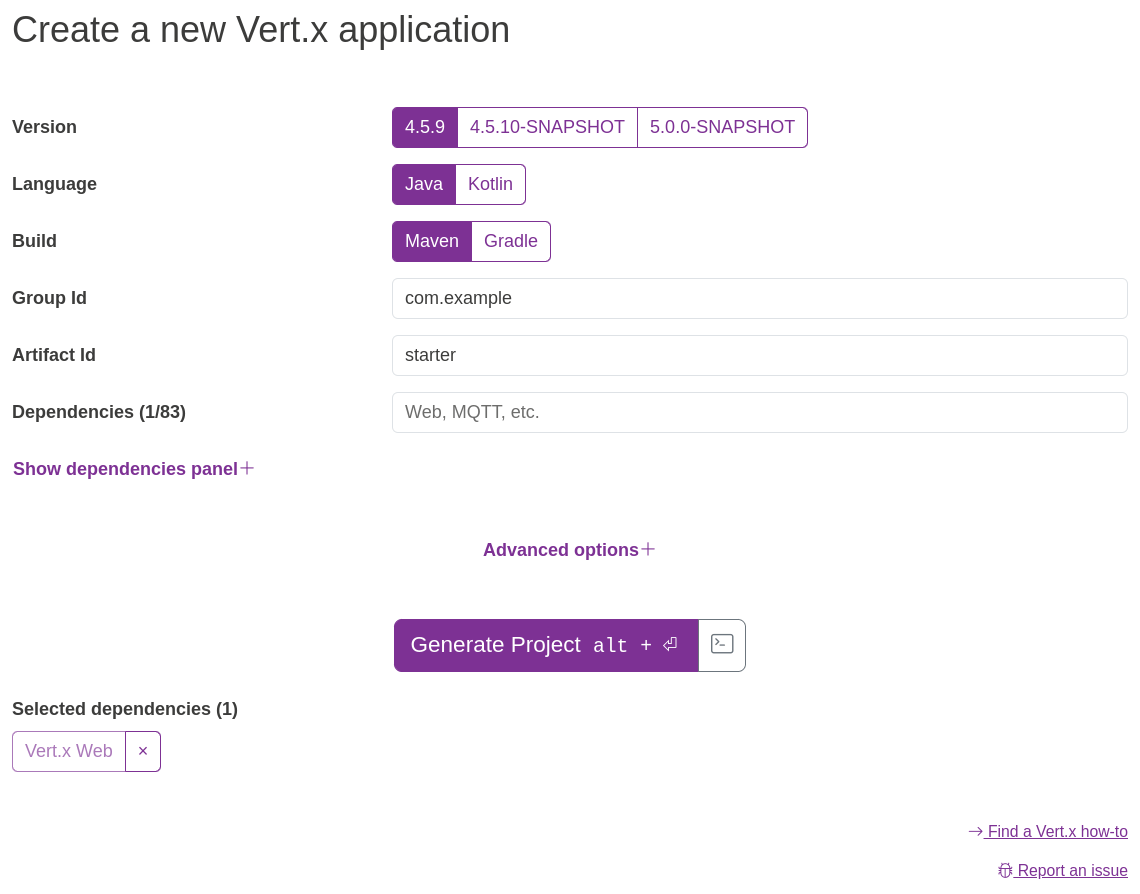
Choose the version of Vert.x you want to use, choose Java as the language, Maven as the build tool, and type the group id and artifact id you want. Then, add Vert.x Web as a dependency by typing it in the “Dependencies” text box. When you’re done, hit the Generate Project button. Save the zip on your computer and unzip it in a folder of your choice.
The generated project contains:
- The Maven build descriptor
pom.xml
configured to build and run your application - A sample Verticle and a sample test using JUnit 5
- An editor configuration to enforce code style
- A Git configuration to ignore files
If you want to try it now, you can download this sample project using Maven or using Gradle.
2Code
Open the project in the editor of your choice and navigate to src/main/java/com/example/starter/MainVerticle.java
.
This source file contains a sample Verticle
(the Vert.x deployment unit) that starts an HTTP server.
You’re going to modify it to greet whoever performs requests to your server.
Change the code as follows:
package com.example.starter;
import io.vertx.core.AbstractVerticle;
import io.vertx.core.MultiMap;
import io.vertx.core.Promise;
import io.vertx.core.json.JsonObject;
import io.vertx.ext.web.Router;
public class MainVerticle extends AbstractVerticle {
@Override
public void start(Promise<Void> startPromise) throws Exception {
// Create a Router
Router router = Router.router(vertx);
// Mount the handler for all incoming requests at every path and HTTP method
router.route().handler(context -> {
// Get the address of the request
String address = context.request().connection().remoteAddress().toString();
// Get the query parameter "name"
MultiMap queryParams = context.queryParams();
String name = queryParams.contains("name") ? queryParams.get("name") : "unknown";
// Write a json response
context.json(
new JsonObject()
.put("name", name)
.put("address", address)
.put("message", "Hello " + name + " connected from " + address)
);
});
// Create the HTTP server
vertx.createHttpServer()
// Handle every request using the router
.requestHandler(router)
// Start listening
.listen(8888)
// Print the port on success
.onSuccess(server -> {
System.out.println("HTTP server started on port " + server.actualPort());
startPromise.complete();
})
// Print the problem on failure
.onFailure(throwable -> {
throwable.printStackTrace();
startPromise.fail(throwable);
});
}
}
This code creates a Vert.x Web Router (the object used to route HTTP requests to specific request handlers)
and starts an HTTP Server on port 8888
. On each request, it returns a JSON object containing the address of the request, the query parameter name
, and a greeting message.
3Run
To run the code, open a terminal and navigate to your project folder. Build the application as follows:
$ mvn package
Then, run the application:
$ mvn exec:java
HTTP server started on port 8888
apr 03, 2020 11:49:21 AM io.vertx.core.impl.launcher.commands.VertxIsolatedDeployer
INFO: Succeeded in deploying verticle
Now that the server is up and running, try to send a request:
$ http http://localhost:8888
HTTP/1.1 200 OK
content-length: 115
content-type: application/json; charset=utf-8
{
"address": "0:0:0:0:0:0:0:1:32806",
"message": "Hello unknown connected from 0:0:0:0:0:0:0:1:32806",
"name": "unknown"
}
$ http http://localhost:8888\?name\="Francesco"
HTTP/1.1 200 OK
content-length: 119
content-type: application/json; charset=utf-8
{
"address": "0:0:0:0:0:0:0:1:32822",
"message": "Hello Francesco connected from 0:0:0:0:0:0:0:1:32822",
"name": "Francesco"
}
4Go further
Now that you have had a taste of how easy and fun it is to get started with Vert.x, here are a few pointers to help guide you further along your journey: